图书介绍
THINKING IN JAVA 2ND EDITION2025|PDF|Epub|mobi|kindle电子书版本百度云盘下载
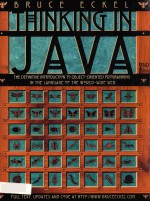
- BRUCE ECKEL 著
- 出版社: PRENTICE HALL
- ISBN:0130273635
- 出版时间:2000
- 标注页数:1130页
- 文件大小:154MB
- 文件页数:1157页
- 主题词:
PDF下载
下载说明
THINKING IN JAVA 2ND EDITIONPDF格式电子书版下载
下载的文件为RAR压缩包。需要使用解压软件进行解压得到PDF格式图书。建议使用BT下载工具Free Download Manager进行下载,简称FDM(免费,没有广告,支持多平台)。本站资源全部打包为BT种子。所以需要使用专业的BT下载软件进行下载。如BitComet qBittorrent uTorrent等BT下载工具。迅雷目前由于本站不是热门资源。不推荐使用!后期资源热门了。安装了迅雷也可以迅雷进行下载!
(文件页数 要大于 标注页数,上中下等多册电子书除外)
注意:本站所有压缩包均有解压码: 点击下载压缩包解压工具
图书目录
Preface1
Preface to the 2nd edition4
Java 26
The CD ROM7
Introduction9
Prerequisites9
Learning Java10
Goals11
Online documentation12
Chapters13
Exercises19
Multimedia CD ROM19
Source code20
Coding standards22
Java versions22
Seminars and mentoring23
Errors23
Note on the cover design24
Acknowledgements25
Internet contributors28
1:Introduction to Objects29
The progress of abstraction30
An object has an interface32
The hidden implementation35
Reusing the implementation37
Inheritance:reusing the interface38
Is-a vs.is-like-a relationships42
Interchangeable objects with polymorphism44
Abstract base classes and interfaces48
Object landscapes and lifetimes49
Collections and iterators51
The singly rooted hierarchy53
Collection libraries and support for easy collection use54
The housekeeping dilemma:who should clean up?55
Exception handling:dealing with errors57
Multithreading58
Persistence60
Java and the Internet60
What is the Web?60
Client-side programming63
Server-side programming70
A separate arena:applications71
Analysis and design71
Phase 0:Make a plan74
Phase 1:What are we making?75
Phase 2:How will we build it?79
Phase 3:Build the core83
Phase 4:Iterate the use cases84
Phase 5:Evolution85
Plans pay off87
Extreme programming88
Write tests first88
Pair programming90
Why Java succeeds91
Systems are easier to91
express and understand91
Maximal leverage with libraries92
Error handling92
Programming in the large92
Strategies for transition93
Guidelines93
Management obstacles95
Java vs.C++?97
Summary98
2:Everything is an Object101
You manipulate objects with references101
You must create all the objects103
Where storage lives103
Special case:primitive types105
Arrays in Java107
You never need to destroy an object107
Scoping108
Scope of objects109
Creating new data types:class110
Fields and methods110
Methods,arguments,and return values112
The argument list114
Building a Java program115
Name visibility115
Using other components116
The static keyword117
Your first Java program119
Compiling and running121
Comments and embedded documentation122
Comment documentation123
Syntax124
Embedded HTML125
@see:referring to other classes125
Class documentation tags126
Variable documentation tags127
Method documentation tags127
Documentation example128
Coding style129
Summary130
Exercises130
3:Controlling Program Flow133
Using Java operators133
Precedence134
Assignment134
Mathematical operators137
Auto increment and decrement139
Relational operators141
Logical operators143
Bitwise operators146
Shift operators147
Ternary if-else operator151
The comma operator152
String operator +153
Common pitfalls when using operators153
Casting operators154
Java has no “sizeof”158
Precedence revisited158
A compendium of operators159
Execution control170
true and false170
if-else171
Iteration172
do-while173
for173
break and continue175
switch183
Summary187
Exercises188
4:Initialization & Cleanup191
Guaranteed initialization with the constructor191
Method overloading194
Distinguishing overloaded methods196
Overloading with primitives197
Overloading on return values202
Default constructors202
The this keyword203
Cleanup:finalization and garbage collection207
What is finalize( ) for?208
You must perform cleanup209
The death condition214
How a garbage collector works215
Member initialization219
Specifying initialization221
Constructor initialization223
Array initialization231
Multidimensional arrays236
Summary239
Exercises240
5:Hiding the Implementation243
package:the library unit244
Creating unique package names247
A custom tool library251
Using imports to change behavior252
Package caveat254
Java access specifiers255
“Friendly”255
public:interface access256
private:you can’t touch that!258
protected:“sort of friendly”260
Interface and implementation261
Class access263
Summary267
Exercises268
6:Reusing Classes271
Composition syntax271
Inheritance syntax275
Initializing the base class278
Combining composition and inheritance281
Guaranteeing proper cleanup283
Name hiding286
Choosing composition vs.inheritance288
protected290
Incremental development291
Upcasting291
Why “upcasting”?293
The final keyword294
Final data294
Final methods299
Final classes301
Final caution302
Initialization and class loading304
Initialization with inheritance304
Summary306
Exercises307
7:Polymorphism311
Upcasting revisited311
Forgetting the object type313
The twist315
Method-call binding315
Producing the right behavior316
Extensibility320
Overriding vs.overloading324
Abstract classes and methods325
Constructors and polymorphism330
Order of constructor calls330
Inheritance and finalize( )333
Behavior of polymorphic methods inside constructors337
Designing with inheritance339
Pure inheritance vs.extension341
Downcasting and run-time type identification343
Summary346
Exercises346
8:Interfaces &Inner Classes349
Interfaces349
“Multiple inheritance” in Java354
Extending an interface with inheritance358
Grouping constants359
Initializing fields in interfaces361
Nesting interfaces362
Inner classes365
Inner classes and upcasting368
Inner classes in methods and scopes370
Anonymous inner classes373
The link to the outer class376
static inner classes379
Referring to the outer class object381
Reaching outward from a multiply-nested class383
Inheriting from inner classes384
Can inner classes be overridden?385
Inner class identifiers387
Why inner classes?388
Inner classes &control frameworks394
Summary402
Exercises403
9:Holding Your Objects407
Arrays407
Arrays are first-class objects409
Returning an array413
The Arrays class415
Filling an array428
Copying an array429
Comparing arrays430
Array element comparisons431
Sorting an array435
Searching a sorted array437
Array summary439
Introduction to containers439
Printing containers441
Filling containers442
Container disadvantage:unknown type450
Sometimes it works anyway452
Making a type-conscious ArrayList454
Iterators456
Container taxonomy460
Collection functionality463
List functionality467
Making a stack om a LinkedList471
Making a queue from a LinkedList472
Set functionality473
SortedSet476
Map functionality476
SortedMap482
Hashing and hash codes482
Overriding hashCode()492
Holding references495
The WeakHashMap498
Iterators revisited500
Choosing an implementation501
Choosing between Lists502
Choosing between Sets506
Choosing between Maps508
Sorting and searching Lists511
Utilities512
Making a Collection or Map unmodiable513
Synchronizing a Collection or Map514
Unsupported operations516
Java 1.0/1.1 containers519
Vector & Enumeration519
Hashtable521
Stack521
BitSet522
Summary524
Exercises525
10:Error Handling with Exceptions531
Basic exceptions532
Exception arguments533
Catching an exception534
The try block535
Exception handlers535
Creating your own exceptions537
The exception specication542
Catching any exception543
Rethrowing an exception545
Standard Java exceptions549
The special case of RuntimeException550
Performing cleanup with finally552
What’s finally for?554
Pitfall:the lost exception557
Exception restrictions558
Constructors562
Exception matching566
Exception guidelines568
Summary568
Exercises569
11:The Java I/O System573
The File class574
A directory lister574
Checking for and creating directories578
Input and output581
Types of InputStream581
Types of OutputStream583
Adding attributes and useful interfaces585
Reading from an InputStream with FilterInputStream586
Writing to an OutputStream with FilterOutputStream587
Readers & Writers589
Sources and sinks of data590
Modifying stream behavior591
Unchanged Classes592
Off by itself:RandomAccessFile593
Typical uses of I/O streams594
Input streams597
Output streams599
A bug?601
Piped streams602
Standard I/O602
Reading from standard input603
Changing System.out to a PrintWriter604
Redirecting standard I/O604
Compression606
Simple compression with GZIP607
Multifile storage with Zip608
Java ARchives (JARs)611
Object serialization613
Finding the class618
Controlling serialization619
Using persistence630
Tokenizing input639
StreamTokenizer639
StringTokenizer642
Checking capitalization style645
Summary655
Exercises656
12:Run-time Type Identification659
The need for RTTI659
The Class object662
Checking before a cast665
RTTI syntax674
Reflection:run-time class information677
A class method extractor679
Summary685
Exercises686
13 Creating Windows & Applets689
The basic applet692
Applet restrictions692
Applet advantages693
Application frameworks694
Running applets inside a Web browser695
Using Appletviewer698
Testing applets698
Running applets from the command line700
A display framework702
Using the Windows Explorer705
Making a button706
Capturing an event707
Text areas711
Controlling layout712
BorderLayout713
FlowLayout714
GridLayout715
GridBagLayout716
Absolute positioning716
BoxLayout717
The best approach?721
The Swing event model722
Event and listener types723
Tracking multiple events730
A catalog of Swing components734
Buttons734
Icons738
Tool tips740
Text fields740
Borders743
JScrollPanes744
A mini-editor747
Check boxes748
Radio buttons750
Combo boxes(drop-down lists)751
List boxes753
Tabbed panes755
Message boxes756
Menus759
Pop-up menus766
Drawing768
Dialog Boxes771
File dialogs776
HTML on Swing components779
Sliders and progress bars780
Trees781
Tables784
Selecting Look & Feel787
The clipboard790
Packaging an applet into a JAR file793
Programming techniques794
Binding events dynamically794
Separating business logic from UI logic796
A canonical form799
Visual programming and Beans800
What is a Bean?801
Extracting BeanInfo with the Introspector804
A more sophisticated Bean811
Packaging a Bean816
More complex Bean support818
More to Beans819
Summary819
Exercises820
14:Multiple Threads825
Responsive user interfaces826
Inheriting from Thread828
Threading for a responsive interface831
Combining the thread with the main class834
Making many threads836
Daemon threads840
Sharing limited resources842
Improperly accessing resources842
How Java shares resources848
JavaBeans revisited854
Blocking859
Becoming blocked860
Deadlock872
Priorities877
Reading and setting priorities878
Thread groups882
Runnable revisited891
Too many threads894
Summary899
Exercises901
15:Distributed Computing903
Network programming904
Identifying a machine905
Sockets909
Serving multiple clients917
Datagrams923
Using URLs from within an applet923
More to networking926
Java Database Connectivity (JDBC)927
Getting the example to work931
A GUI version of the lookup program935
Why the JDBC API seems so complex938
A more sophisticated example939
Servlets948
The basic servlet949
Servlets and multithreading954
Handling sessions with servlets955
Running the servlet examples960
Java Server Pages960
Implicit objects962
JSP directives963
JSP scripting elements964
Extracting fields and values966
JSP page attributes and scope968
Manipulating sessions in JSP969
Creating and modifying cookies971
JSP summary972
RMI (Remote Method Invocation)973
Remote interfaces973
Implementing the remote interface974
Creating stubs and skeletons978
Using the remote object979
CORBA980
CORBA fundamentals981
An example983
Java Applets and CORBA989
CORBA vs.RMI989
Enterprise JavaBeans990
JavaBeans vs.EJBs991
The EJB specification992
EJB components993
The pieces of an EJB component994
EJB operation995
Types of EJBs996
Developing an EJB997
EJB summary1003
Jini:distributed services1003
Jini in context1003
What is Jini?1004
How Jini works1005
The discovery process1006
The join process1006
The lookup process1007
Separation of interface and implementation1008
Abstracting distributed systems1009
Summary1010
Exercises1010
A:Passing &Returning Objects1013
Passing references around1014
Aliasing1014
Making local copies1017
Pass by value1018
Cloning objects1018
Adding cloneability to a class1020
Successful cloning1022
The effect of Object.clone()1025
Cloning a composed object1027
A deep copy with ArrayList1030
Deep copy via serialization1032
Adding cloneabilityfurther down a hierarchy1034
Why this strange design?1035
Controlling cloneability1036
The copy constructor1042
Read-only classes1047
Creating read-only classes1049
The drawback to immutability1050
Immutable Strings1052
The String and StringBuffer classes1056
Strings are special1060
Summary1060
Exercises1062
B :The Java Native Interface (JNI)1065
Calling a native method1066
The header file generator:javah1067
Name mangling and function signatures1068
Implementing your DLL1068
Accessing JNI functions:the JNIEnv argument1069
Accessing Java Strings1071
Passing and using Java objects1071
JNI and Java exceptions1074
JNI and threading1075
Using a preexisting code base1075
Additional information1076
C:Java Programming Guidelines1077
Design1077
Implementation1084
D:Resources1091
Software1091
Books1091
Analysis & design1093
Python1095
My own list of books1096
Index1099
热门推荐
- 869735.html
- 2626268.html
- 3241399.html
- 1650529.html
- 1508901.html
- 2247610.html
- 3728669.html
- 2436630.html
- 2105427.html
- 1632037.html
- http://www.ickdjs.cc/book_3307673.html
- http://www.ickdjs.cc/book_3377894.html
- http://www.ickdjs.cc/book_1004291.html
- http://www.ickdjs.cc/book_3165082.html
- http://www.ickdjs.cc/book_1600340.html
- http://www.ickdjs.cc/book_1633275.html
- http://www.ickdjs.cc/book_3504376.html
- http://www.ickdjs.cc/book_857806.html
- http://www.ickdjs.cc/book_2676530.html
- http://www.ickdjs.cc/book_810774.html